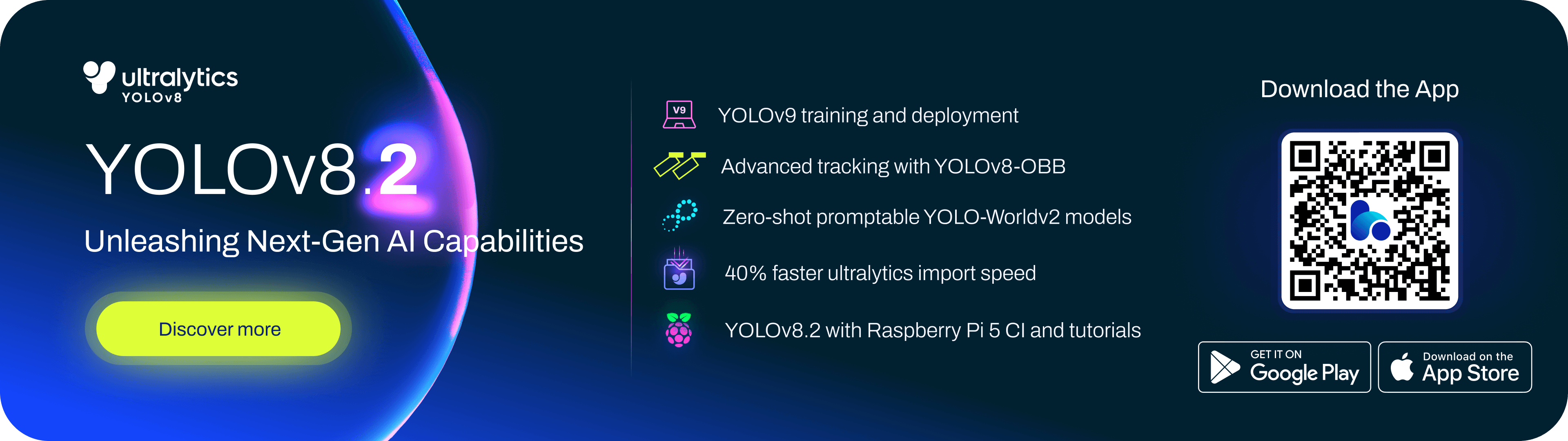
中文 | 한국어 | 日本語 | Русский | Deutsch | Français | Español | Português | Türkçe | Tiếng Việt | हिन्दी | العربية
Welcome to the Ultralytics YOLOv8 🚀 notebook! YOLOv8 is the latest version of the YOLO (You Only Look Once) AI models developed by Ultralytics. This notebook serves as the starting point for exploring the various resources available to help you get started with YOLOv8 and understand its features and capabilities.
YOLOv8 models are fast, accurate, and easy to use, making them ideal for various object detection and image segmentation tasks. They can be trained on large datasets and run on diverse hardware platforms, from CPUs to GPUs.
We hope that the resources in this notebook will help you get the most out of YOLOv8. Please browse the YOLOv8 Docs for details, raise an issue on GitHub for support, and join our Discord community for questions and discussions!
Setup#
Pip install ultralytics
and dependencies and check software and hardware.
%pip install ultralytics
import ultralytics
ultralytics.checks()
1. Predict#
YOLOv8 may be used directly in the Command Line Interface (CLI) with a yolo
command for a variety of tasks and modes and accepts additional arguments, i.e. imgsz=640
. See a full list of available yolo
arguments and other details in the YOLOv8 Predict Docs.
# Run inference on an image with YOLOv8n
!yolo predict model=yolov8n.pt source='https://ultralytics.com/images/zidane.jpg'
2. Val#
Validate a model’s accuracy on the COCO dataset’s val
or test
splits. The latest YOLOv8 models are downloaded automatically the first time they are used. See YOLOv8 Val Docs for more information.
# Download COCO val
import torch
torch.hub.download_url_to_file('https://ultralytics.com/assets/coco2017val.zip', 'tmp.zip') # download (780M - 5000 images)
!unzip -q tmp.zip -d datasets && rm tmp.zip # unzip
# Validate YOLOv8n on COCO8 val
!yolo val model=yolov8n.pt data=coco8.yaml
3. Train#
Train YOLOv8 on Detect, Segment, Classify and Pose datasets. See YOLOv8 Train Docs for more information.
#@title Select YOLOv8 🚀 logger {run: 'auto'}
logger = 'Comet' #@param ['Comet', 'TensorBoard']
if logger == 'Comet':
%pip install -q comet_ml
import comet_ml; comet_ml.init()
elif logger == 'TensorBoard':
%load_ext tensorboard
%tensorboard --logdir .
# Train YOLOv8n on COCO8 for 3 epochs
!yolo train model=yolov8n.pt data=coco8.yaml epochs=3 imgsz=640
4. Export#
Export a YOLOv8 model to any supported format below with the format
argument, i.e. format=onnx
. See YOLOv8 Export Docs for more information.
💡 ProTip: Export to ONNX or OpenVINO for up to 3x CPU speedup.
💡 ProTip: Export to TensorRT for up to 5x GPU speedup.
Format |
|
Model |
Metadata |
Arguments |
---|---|---|---|---|
- |
|
✅ |
- |
|
|
|
✅ |
|
|
|
|
✅ |
|
|
|
|
✅ |
|
|
|
|
✅ |
|
|
|
|
✅ |
|
|
|
|
✅ |
|
|
|
|
❌ |
|
|
|
|
✅ |
|
|
|
|
✅ |
|
|
|
|
✅ |
|
|
|
|
✅ |
|
|
|
|
✅ |
|
!yolo export model=yolov8n.pt format=torchscript
5. Python Usage#
YOLOv8 was reimagined using Python-first principles for the most seamless Python YOLO experience yet. YOLOv8 models can be loaded from a trained checkpoint or created from scratch. Then methods are used to train, val, predict, and export the model. See detailed Python usage examples in the YOLOv8 Python Docs.
import sys
# sys.path.append("/media/pc/data/lxw/BaiduNetdiskDownload/电信N合一算法模型评估/product_models/vehicle/model2out/det_traffic")
sys.path.append("/media/pc/data/lxw/ai/ultralytics")
from ultralytics import YOLO
# Load a model
model = YOLO('yolov8n.yaml') # build a new model from scratch
model = YOLO('yolov8n.pt') # load a pretrained model (recommended for training)
# Use the model
results = model.train(data='coco8.yaml', epochs=3) # train the model
results = model.val() # evaluate model performance on the validation set
results = model('https://ultralytics.com/images/bus.jpg') # predict on an image
results = model.export(format='onnx') # export the model to ONNX format
Ultralytics YOLOv8.2.48 🚀 Python-3.12.3 torch-2.3.0 CUDA:0 (NVIDIA GeForce RTX 3090, 24037MiB)
engine/trainer: task=detect, mode=train, model=yolov8n.pt, data=coco8.yaml, epochs=3, time=None, patience=100, batch=16, imgsz=640, save=True, save_period=-1, cache=False, device=None, workers=8, project=None, name=train3, exist_ok=False, pretrained=True, optimizer=auto, verbose=True, seed=0, deterministic=True, single_cls=False, rect=False, cos_lr=False, close_mosaic=10, resume=False, amp=True, fraction=1.0, profile=False, freeze=None, multi_scale=False, overlap_mask=True, mask_ratio=4, dropout=0.0, val=True, split=val, save_json=False, save_hybrid=False, conf=None, iou=0.7, max_det=300, half=False, dnn=False, plots=True, source=None, vid_stride=1, stream_buffer=False, visualize=False, augment=False, agnostic_nms=False, classes=None, retina_masks=False, embed=None, show=False, save_frames=False, save_txt=False, save_conf=False, save_crop=False, show_labels=True, show_conf=True, show_boxes=True, line_width=None, format=torchscript, keras=False, optimize=False, int8=False, dynamic=False, simplify=False, opset=None, workspace=4, nms=False, lr0=0.01, lrf=0.01, momentum=0.937, weight_decay=0.0005, warmup_epochs=3.0, warmup_momentum=0.8, warmup_bias_lr=0.1, box=7.5, cls=0.5, dfl=1.5, pose=12.0, kobj=1.0, label_smoothing=0.0, nbs=64, hsv_h=0.015, hsv_s=0.7, hsv_v=0.4, degrees=0.0, translate=0.1, scale=0.5, shear=0.0, perspective=0.0, flipud=0.0, fliplr=0.5, bgr=0.0, mosaic=1.0, mixup=0.0, copy_paste=0.0, auto_augment=randaugment, erasing=0.4, crop_fraction=1.0, cfg=None, tracker=botsort.yaml, save_dir=/media/pc/data/board/arria10/lxw/tasks/tools/npu_user_demos/runs/detect/train3
from n params module arguments
0 -1 1 464 ultralytics.nn.modules.conv.Conv [3, 16, 3, 2]
1 -1 1 4672 ultralytics.nn.modules.conv.Conv [16, 32, 3, 2]
2 -1 1 7360 ultralytics.nn.modules.block.C2f [32, 32, 1, True]
3 -1 1 18560 ultralytics.nn.modules.conv.Conv [32, 64, 3, 2]
4 -1 2 49664 ultralytics.nn.modules.block.C2f [64, 64, 2, True]
5 -1 1 73984 ultralytics.nn.modules.conv.Conv [64, 128, 3, 2]
6 -1 2 197632 ultralytics.nn.modules.block.C2f [128, 128, 2, True]
7 -1 1 295424 ultralytics.nn.modules.conv.Conv [128, 256, 3, 2]
8 -1 1 460288 ultralytics.nn.modules.block.C2f [256, 256, 1, True]
9 -1 1 164608 ultralytics.nn.modules.block.SPPF [256, 256, 5]
10 -1 1 0 torch.nn.modules.upsampling.Upsample [None, 2, 'nearest']
11 [-1, 6] 1 0 ultralytics.nn.modules.conv.Concat [1]
12 -1 1 148224 ultralytics.nn.modules.block.C2f [384, 128, 1]
13 -1 1 0 torch.nn.modules.upsampling.Upsample [None, 2, 'nearest']
14 [-1, 4] 1 0 ultralytics.nn.modules.conv.Concat [1]
15 -1 1 37248 ultralytics.nn.modules.block.C2f [192, 64, 1]
16 -1 1 36992 ultralytics.nn.modules.conv.Conv [64, 64, 3, 2]
17 [-1, 12] 1 0 ultralytics.nn.modules.conv.Concat [1]
18 -1 1 123648 ultralytics.nn.modules.block.C2f [192, 128, 1]
19 -1 1 147712 ultralytics.nn.modules.conv.Conv [128, 128, 3, 2]
20 [-1, 9] 1 0 ultralytics.nn.modules.conv.Concat [1]
21 -1 1 493056 ultralytics.nn.modules.block.C2f [384, 256, 1]
22 [15, 18, 21] 1 897664 ultralytics.nn.modules.head.Detect [80, [64, 128, 256]]
Model summary: 225 layers, 3157200 parameters, 3157184 gradients
Transferred 355/355 items from pretrained weights
Freezing layer 'model.22.dfl.conv.weight'
AMP: running Automatic Mixed Precision (AMP) checks with YOLOv8n...
WARNING ⚠️ NMS time limit 2.050s exceeded
AMP: checks passed ✅
Plotting labels to /media/pc/data/board/arria10/lxw/tasks/tools/npu_user_demos/runs/detect/train3/labels.jpg...
optimizer: 'optimizer=auto' found, ignoring 'lr0=0.01' and 'momentum=0.937' and determining best 'optimizer', 'lr0' and 'momentum' automatically...
optimizer: AdamW(lr=0.000119, momentum=0.9) with parameter groups 57 weight(decay=0.0), 64 weight(decay=0.0005), 63 bias(decay=0.0)
Image sizes 640 train, 640 val
Using 8 dataloader workers
Logging results to /media/pc/data/board/arria10/lxw/tasks/tools/npu_user_demos/runs/detect/train3
Starting training for 3 epochs...
Epoch GPU_mem box_loss cls_loss dfl_loss Instances Size
all 4 17 0.62 0.878 0.888 0.612
Epoch GPU_mem box_loss cls_loss dfl_loss Instances Size
all 4 17 0.597 0.897 0.888 0.623
Epoch GPU_mem box_loss cls_loss dfl_loss Instances Size
all 4 17 0.57 0.833 0.872 0.62
3 epochs completed in 0.001 hours.
Optimizer stripped from /media/pc/data/board/arria10/lxw/tasks/tools/npu_user_demos/runs/detect/train3/weights/last.pt, 6.5MB
Optimizer stripped from /media/pc/data/board/arria10/lxw/tasks/tools/npu_user_demos/runs/detect/train3/weights/best.pt, 6.5MB
Validating /media/pc/data/board/arria10/lxw/tasks/tools/npu_user_demos/runs/detect/train3/weights/best.pt...
Ultralytics YOLOv8.2.48 🚀 Python-3.12.3 torch-2.3.0 CUDA:0 (NVIDIA GeForce RTX 3090, 24037MiB)
Model summary (fused): 168 layers, 3151904 parameters, 0 gradients
all 4 17 0.598 0.898 0.888 0.623
person 3 10 0.644 0.5 0.52 0.286
dog 1 1 0.32 1 0.995 0.597
horse 1 2 0.699 1 0.995 0.648
elephant 1 2 0.629 0.886 0.828 0.319
umbrella 1 1 0.541 1 0.995 0.995
potted plant 1 1 0.758 1 0.995 0.895
Speed: 0.3ms preprocess, 2.8ms inference, 0.0ms loss, 1.3ms postprocess per image
Results saved to /media/pc/data/board/arria10/lxw/tasks/tools/npu_user_demos/runs/detect/train3
Ultralytics YOLOv8.2.48 🚀 Python-3.12.3 torch-2.3.0 CUDA:0 (NVIDIA GeForce RTX 3090, 24037MiB)
Model summary (fused): 168 layers, 3151904 parameters, 0 gradients
all 4 17 0.598 0.897 0.888 0.625
person 3 10 0.644 0.5 0.519 0.296
dog 1 1 0.319 1 0.995 0.597
horse 1 2 0.7 1 0.995 0.648
elephant 1 2 0.627 0.881 0.828 0.319
umbrella 1 1 0.541 1 0.995 0.995
potted plant 1 1 0.758 1 0.995 0.895
Speed: 0.3ms preprocess, 64.9ms inference, 0.0ms loss, 1.9ms postprocess per image
Results saved to /media/pc/data/board/arria10/lxw/tasks/tools/npu_user_demos/runs/detect/train32
Found https://ultralytics.com/images/bus.jpg locally at bus.jpg
image 1/1 /media/pc/data/lxw/ai/tvm-book/tests/book/doc/tests/bus.jpg: 640x480 4 persons, 1 bus, 1 stop sign, 17.1ms
Speed: 2.7ms preprocess, 17.1ms inference, 2.5ms postprocess per image at shape (1, 3, 640, 480)
Ultralytics YOLOv8.2.48 🚀 Python-3.12.3 torch-2.3.0 CPU (Intel Xeon E5-2678 v3 2.50GHz)
PyTorch: starting from '/media/pc/data/board/arria10/lxw/tasks/tools/npu_user_demos/runs/detect/train3/weights/best.pt' with input shape (1, 3, 640, 640) BCHW and output shape(s) (1, 84, 8400) (6.2 MB)
ONNX: starting export with onnx 1.16.1 opset 17...
ONNX: export success ✅ 0.9s, saved as '/media/pc/data/board/arria10/lxw/tasks/tools/npu_user_demos/runs/detect/train3/weights/best.onnx' (12.2 MB)
Export complete (2.5s)
Results saved to /media/pc/data/board/arria10/lxw/tasks/tools/npu_user_demos/runs/detect/train3/weights
Predict: yolo predict task=detect model=/media/pc/data/board/arria10/lxw/tasks/tools/npu_user_demos/runs/detect/train3/weights/best.onnx imgsz=640
Validate: yolo val task=detect model=/media/pc/data/board/arria10/lxw/tasks/tools/npu_user_demos/runs/detect/train3/weights/best.onnx imgsz=640 data=/media/pc/data/lxw/ai/ultralytics/ultralytics/cfg/datasets/coco8.yaml
Visualize: https://netron.app
/media/pc/data/tmp/cache/conda/envs/py312x/lib/python3.12/site-packages/torch/nn/modules/conv.py:456: UserWarning: Plan failed with a cudnnException: CUDNN_BACKEND_EXECUTION_PLAN_DESCRIPTOR: cudnnFinalize Descriptor Failed cudnn_status: CUDNN_STATUS_NOT_SUPPORTED (Triggered internally at /opt/conda/conda-bld/pytorch_1712608847532/work/aten/src/ATen/native/cudnn/Conv_v8.cpp:919.)
return F.conv2d(input, weight, bias, self.stride,
train: Scanning /media/pc/data/board/arria10/lxw/tasks/tools/datasets/coco8/labels/train.cache... 4 images, 0 backgrounds, 0 corrupt: 100%|██████████| 4/4 [00:00<?, ?it/s]
val: Scanning /media/pc/data/board/arria10/lxw/tasks/tools/datasets/coco8/labels/val.cache... 4 images, 0 backgrounds, 0 corrupt: 100%|██████████| 4/4 [00:00<?, ?it/s]
0%| | 0/1 [00:00<?, ?it/s]/media/pc/data/tmp/cache/conda/envs/py312x/lib/python3.12/site-packages/torch/nn/modules/conv.py:456: UserWarning: Plan failed with a cudnnException: CUDNN_BACKEND_EXECUTION_PLAN_DESCRIPTOR: cudnnFinalize Descriptor Failed cudnn_status: CUDNN_STATUS_NOT_SUPPORTED (Triggered internally at /opt/conda/conda-bld/pytorch_1712608847532/work/aten/src/ATen/native/cudnn/Conv_v8.cpp:919.)
return F.conv2d(input, weight, bias, self.stride,
1/3 0.822G 1.069 3.51 1.514 21 640: 100%|██████████| 1/1 [00:01<00:00, 1.34s/it]
Class Images Instances Box(P R mAP50 mAP50-95): 100%|██████████| 1/1 [00:00<00:00, 2.78it/s]
2/3 0.818G 1.132 2.784 1.44 36 640: 100%|██████████| 1/1 [00:00<00:00, 8.43it/s]
Class Images Instances Box(P R mAP50 mAP50-95): 100%|██████████| 1/1 [00:00<00:00, 17.42it/s]
3/3 0.818G 1.02 2.124 1.266 20 640: 100%|██████████| 1/1 [00:00<00:00, 8.89it/s]
Class Images Instances Box(P R mAP50 mAP50-95): 100%|██████████| 1/1 [00:00<00:00, 17.75it/s]
/media/pc/data/tmp/cache/conda/envs/py312x/lib/python3.12/site-packages/torch/nn/modules/conv.py:456: UserWarning: Plan failed with a cudnnException: CUDNN_BACKEND_EXECUTION_PLAN_DESCRIPTOR: cudnnFinalize Descriptor Failed cudnn_status: CUDNN_STATUS_NOT_SUPPORTED (Triggered internally at /opt/conda/conda-bld/pytorch_1712608847532/work/aten/src/ATen/native/cudnn/Conv_v8.cpp:919.)
return F.conv2d(input, weight, bias, self.stride,
Class Images Instances Box(P R mAP50 mAP50-95): 100%|██████████| 1/1 [00:00<00:00, 25.77it/s]
val: Scanning /media/pc/data/board/arria10/lxw/tasks/tools/datasets/coco8/labels/val.cache... 4 images, 0 backgrounds, 0 corrupt: 100%|██████████| 4/4 [00:00<?, ?it/s]
Class Images Instances Box(P R mAP50 mAP50-95): 100%|██████████| 1/1 [00:00<00:00, 2.88it/s]
6. Tasks#
YOLOv8 can train, val, predict and export models for the most common tasks in vision AI: Detect, Segment, Classify and Pose. See YOLOv8 Tasks Docs for more information.
1. Detection#
YOLOv8 detection models have no suffix and are the default YOLOv8 models, i.e. yolov8n.pt
and are pretrained on COCO. See Detection Docs for full details.
# Load YOLOv8n, train it on COCO128 for 3 epochs and predict an image with it
from ultralytics import YOLO
model = YOLO('yolov8n.pt') # load a pretrained YOLOv8n detection model
model.train(data='coco8.yaml', epochs=3) # train the model
model('https://ultralytics.com/images/bus.jpg') # predict on an image
Ultralytics YOLOv8.2.48 🚀 Python-3.12.3 torch-2.3.0 CUDA:0 (NVIDIA GeForce RTX 3090, 24037MiB)
engine/trainer: task=detect, mode=train, model=yolov8n.pt, data=coco8.yaml, epochs=3, time=None, patience=100, batch=16, imgsz=640, save=True, save_period=-1, cache=False, device=None, workers=8, project=None, name=train4, exist_ok=False, pretrained=True, optimizer=auto, verbose=True, seed=0, deterministic=True, single_cls=False, rect=False, cos_lr=False, close_mosaic=10, resume=False, amp=True, fraction=1.0, profile=False, freeze=None, multi_scale=False, overlap_mask=True, mask_ratio=4, dropout=0.0, val=True, split=val, save_json=False, save_hybrid=False, conf=None, iou=0.7, max_det=300, half=False, dnn=False, plots=True, source=None, vid_stride=1, stream_buffer=False, visualize=False, augment=False, agnostic_nms=False, classes=None, retina_masks=False, embed=None, show=False, save_frames=False, save_txt=False, save_conf=False, save_crop=False, show_labels=True, show_conf=True, show_boxes=True, line_width=None, format=torchscript, keras=False, optimize=False, int8=False, dynamic=False, simplify=False, opset=None, workspace=4, nms=False, lr0=0.01, lrf=0.01, momentum=0.937, weight_decay=0.0005, warmup_epochs=3.0, warmup_momentum=0.8, warmup_bias_lr=0.1, box=7.5, cls=0.5, dfl=1.5, pose=12.0, kobj=1.0, label_smoothing=0.0, nbs=64, hsv_h=0.015, hsv_s=0.7, hsv_v=0.4, degrees=0.0, translate=0.1, scale=0.5, shear=0.0, perspective=0.0, flipud=0.0, fliplr=0.5, bgr=0.0, mosaic=1.0, mixup=0.0, copy_paste=0.0, auto_augment=randaugment, erasing=0.4, crop_fraction=1.0, cfg=None, tracker=botsort.yaml, save_dir=/media/pc/data/board/arria10/lxw/tasks/tools/npu_user_demos/runs/detect/train4
from n params module arguments
0 -1 1 464 ultralytics.nn.modules.conv.Conv [3, 16, 3, 2]
1 -1 1 4672 ultralytics.nn.modules.conv.Conv [16, 32, 3, 2]
2 -1 1 7360 ultralytics.nn.modules.block.C2f [32, 32, 1, True]
3 -1 1 18560 ultralytics.nn.modules.conv.Conv [32, 64, 3, 2]
4 -1 2 49664 ultralytics.nn.modules.block.C2f [64, 64, 2, True]
5 -1 1 73984 ultralytics.nn.modules.conv.Conv [64, 128, 3, 2]
6 -1 2 197632 ultralytics.nn.modules.block.C2f [128, 128, 2, True]
7 -1 1 295424 ultralytics.nn.modules.conv.Conv [128, 256, 3, 2]
8 -1 1 460288 ultralytics.nn.modules.block.C2f [256, 256, 1, True]
9 -1 1 164608 ultralytics.nn.modules.block.SPPF [256, 256, 5]
10 -1 1 0 torch.nn.modules.upsampling.Upsample [None, 2, 'nearest']
11 [-1, 6] 1 0 ultralytics.nn.modules.conv.Concat [1]
12 -1 1 148224 ultralytics.nn.modules.block.C2f [384, 128, 1]
13 -1 1 0 torch.nn.modules.upsampling.Upsample [None, 2, 'nearest']
14 [-1, 4] 1 0 ultralytics.nn.modules.conv.Concat [1]
15 -1 1 37248 ultralytics.nn.modules.block.C2f [192, 64, 1]
16 -1 1 36992 ultralytics.nn.modules.conv.Conv [64, 64, 3, 2]
17 [-1, 12] 1 0 ultralytics.nn.modules.conv.Concat [1]
18 -1 1 123648 ultralytics.nn.modules.block.C2f [192, 128, 1]
19 -1 1 147712 ultralytics.nn.modules.conv.Conv [128, 128, 3, 2]
20 [-1, 9] 1 0 ultralytics.nn.modules.conv.Concat [1]
21 -1 1 493056 ultralytics.nn.modules.block.C2f [384, 256, 1]
22 [15, 18, 21] 1 897664 ultralytics.nn.modules.head.Detect [80, [64, 128, 256]]
Model summary: 225 layers, 3157200 parameters, 3157184 gradients
Transferred 355/355 items from pretrained weights
Freezing layer 'model.22.dfl.conv.weight'
AMP: running Automatic Mixed Precision (AMP) checks with YOLOv8n...
AMP: checks passed ✅
Plotting labels to /media/pc/data/board/arria10/lxw/tasks/tools/npu_user_demos/runs/detect/train4/labels.jpg...
optimizer: 'optimizer=auto' found, ignoring 'lr0=0.01' and 'momentum=0.937' and determining best 'optimizer', 'lr0' and 'momentum' automatically...
optimizer: AdamW(lr=0.000119, momentum=0.9) with parameter groups 57 weight(decay=0.0), 64 weight(decay=0.0005), 63 bias(decay=0.0)
Image sizes 640 train, 640 val
Using 8 dataloader workers
Logging results to /media/pc/data/board/arria10/lxw/tasks/tools/npu_user_demos/runs/detect/train4
Starting training for 3 epochs...
Epoch GPU_mem box_loss cls_loss dfl_loss Instances Size
all 4 17 0.62 0.878 0.888 0.612
Epoch GPU_mem box_loss cls_loss dfl_loss Instances Size
all 4 17 0.597 0.897 0.888 0.623
Epoch GPU_mem box_loss cls_loss dfl_loss Instances Size
all 4 17 0.57 0.833 0.872 0.62
3 epochs completed in 0.001 hours.
Optimizer stripped from /media/pc/data/board/arria10/lxw/tasks/tools/npu_user_demos/runs/detect/train4/weights/last.pt, 6.5MB
Optimizer stripped from /media/pc/data/board/arria10/lxw/tasks/tools/npu_user_demos/runs/detect/train4/weights/best.pt, 6.5MB
Validating /media/pc/data/board/arria10/lxw/tasks/tools/npu_user_demos/runs/detect/train4/weights/best.pt...
Ultralytics YOLOv8.2.48 🚀 Python-3.12.3 torch-2.3.0 CUDA:0 (NVIDIA GeForce RTX 3090, 24037MiB)
Model summary (fused): 168 layers, 3151904 parameters, 0 gradients
all 4 17 0.598 0.898 0.888 0.623
person 3 10 0.644 0.5 0.52 0.286
dog 1 1 0.32 1 0.995 0.597
horse 1 2 0.699 1 0.995 0.648
elephant 1 2 0.629 0.886 0.828 0.319
umbrella 1 1 0.541 1 0.995 0.995
potted plant 1 1 0.758 1 0.995 0.895
Speed: 0.2ms preprocess, 2.4ms inference, 0.0ms loss, 1.1ms postprocess per image
Results saved to /media/pc/data/board/arria10/lxw/tasks/tools/npu_user_demos/runs/detect/train4
Found https://ultralytics.com/images/bus.jpg locally at bus.jpg
image 1/1 /media/pc/data/lxw/ai/tvm-book/tests/book/doc/tests/bus.jpg: 640x480 4 persons, 1 bus, 1 stop sign, 9.6ms
Speed: 2.7ms preprocess, 9.6ms inference, 2.0ms postprocess per image at shape (1, 3, 640, 480)
train: Scanning /media/pc/data/board/arria10/lxw/tasks/tools/datasets/coco8/labels/train.cache... 4 images, 0 backgrounds, 0 corrupt: 100%|██████████| 4/4 [00:00<?, ?it/s]
val: Scanning /media/pc/data/board/arria10/lxw/tasks/tools/datasets/coco8/labels/val.cache... 4 images, 0 backgrounds, 0 corrupt: 100%|██████████| 4/4 [00:00<?, ?it/s]
1/3 0.824G 1.069 3.51 1.514 21 640: 100%|██████████| 1/1 [00:00<00:00, 6.12it/s]
Class Images Instances Box(P R mAP50 mAP50-95): 100%|██████████| 1/1 [00:00<00:00, 13.47it/s]
2/3 0.818G 1.132 2.784 1.44 36 640: 100%|██████████| 1/1 [00:00<00:00, 8.45it/s]
Class Images Instances Box(P R mAP50 mAP50-95): 100%|██████████| 1/1 [00:00<00:00, 17.52it/s]
3/3 0.818G 1.02 2.124 1.266 20 640: 100%|██████████| 1/1 [00:00<00:00, 8.75it/s]
Class Images Instances Box(P R mAP50 mAP50-95): 100%|██████████| 1/1 [00:00<00:00, 17.54it/s]
Class Images Instances Box(P R mAP50 mAP50-95): 100%|██████████| 1/1 [00:00<00:00, 31.84it/s]
[ultralytics.engine.results.Results object with attributes:
boxes: ultralytics.engine.results.Boxes object
keypoints: None
masks: None
names: {0: 'person', 1: 'bicycle', 2: 'car', 3: 'motorcycle', 4: 'airplane', 5: 'bus', 6: 'train', 7: 'truck', 8: 'boat', 9: 'traffic light', 10: 'fire hydrant', 11: 'stop sign', 12: 'parking meter', 13: 'bench', 14: 'bird', 15: 'cat', 16: 'dog', 17: 'horse', 18: 'sheep', 19: 'cow', 20: 'elephant', 21: 'bear', 22: 'zebra', 23: 'giraffe', 24: 'backpack', 25: 'umbrella', 26: 'handbag', 27: 'tie', 28: 'suitcase', 29: 'frisbee', 30: 'skis', 31: 'snowboard', 32: 'sports ball', 33: 'kite', 34: 'baseball bat', 35: 'baseball glove', 36: 'skateboard', 37: 'surfboard', 38: 'tennis racket', 39: 'bottle', 40: 'wine glass', 41: 'cup', 42: 'fork', 43: 'knife', 44: 'spoon', 45: 'bowl', 46: 'banana', 47: 'apple', 48: 'sandwich', 49: 'orange', 50: 'broccoli', 51: 'carrot', 52: 'hot dog', 53: 'pizza', 54: 'donut', 55: 'cake', 56: 'chair', 57: 'couch', 58: 'potted plant', 59: 'bed', 60: 'dining table', 61: 'toilet', 62: 'tv', 63: 'laptop', 64: 'mouse', 65: 'remote', 66: 'keyboard', 67: 'cell phone', 68: 'microwave', 69: 'oven', 70: 'toaster', 71: 'sink', 72: 'refrigerator', 73: 'book', 74: 'clock', 75: 'vase', 76: 'scissors', 77: 'teddy bear', 78: 'hair drier', 79: 'toothbrush'}
obb: None
orig_img: array([[[122, 148, 172],
[120, 146, 170],
[125, 153, 177],
...,
[157, 170, 184],
[158, 171, 185],
[158, 171, 185]],
[[127, 153, 177],
[124, 150, 174],
[127, 155, 179],
...,
[158, 171, 185],
[159, 172, 186],
[159, 172, 186]],
[[128, 154, 178],
[126, 152, 176],
[126, 154, 178],
...,
[158, 171, 185],
[158, 171, 185],
[158, 171, 185]],
...,
[[185, 185, 191],
[182, 182, 188],
[179, 179, 185],
...,
[114, 107, 112],
[115, 105, 111],
[116, 106, 112]],
[[157, 157, 163],
[180, 180, 186],
[185, 186, 190],
...,
[107, 97, 103],
[102, 92, 98],
[108, 98, 104]],
[[112, 112, 118],
[160, 160, 166],
[169, 170, 174],
...,
[ 99, 89, 95],
[ 96, 86, 92],
[102, 92, 98]]], dtype=uint8)
orig_shape: (1080, 810)
path: '/media/pc/data/lxw/ai/tvm-book/tests/book/doc/tests/bus.jpg'
probs: None
save_dir: '/media/pc/data/board/arria10/lxw/tasks/tools/npu_user_demos/runs/detect/train42'
speed: {'preprocess': 2.669095993041992, 'inference': 9.616613388061523, 'postprocess': 1.987457275390625}]
2. Segmentation#
YOLOv8 segmentation models use the -seg
suffix, i.e. yolov8n-seg.pt
and are pretrained on COCO. See Segmentation Docs for full details.
# Load YOLOv8n-seg, train it on COCO128-seg for 3 epochs and predict an image with it
from ultralytics import YOLO
model = YOLO('yolov8n-seg.pt') # load a pretrained YOLOv8n segmentation model
model.train(data='coco8-seg.yaml', epochs=3) # train the model
model('https://ultralytics.com/images/bus.jpg') # predict on an image
3. Classification#
YOLOv8 classification models use the -cls
suffix, i.e. yolov8n-cls.pt
and are pretrained on ImageNet. See Classification Docs for full details.
# Load YOLOv8n-cls, train it on mnist160 for 3 epochs and predict an image with it
from ultralytics import YOLO
model = YOLO('yolov8n-cls.pt') # load a pretrained YOLOv8n classification model
model.train(data='mnist160', epochs=3) # train the model
model('https://ultralytics.com/images/bus.jpg') # predict on an image
4. Pose#
YOLOv8 pose models use the -pose
suffix, i.e. yolov8n-pose.pt
and are pretrained on COCO Keypoints. See Pose Docs for full details.
# Load YOLOv8n-pose, train it on COCO8-pose for 3 epochs and predict an image with it
from ultralytics import YOLO
model = YOLO('yolov8n-pose.pt') # load a pretrained YOLOv8n pose model
model.train(data='coco8-pose.yaml', epochs=3) # train the model
model('https://ultralytics.com/images/bus.jpg') # predict on an image
4. Oriented Bounding Boxes (OBB)#
YOLOv8 OBB models use the -obb
suffix, i.e. yolov8n-obb.pt
and are pretrained on the DOTA dataset. See OBB Docs for full details.
# Load YOLOv8n-obb, train it on DOTA8 for 3 epochs and predict an image with it
from ultralytics import YOLO
model = YOLO('yolov8n-obb.pt') # load a pretrained YOLOv8n OBB model
model.train(data='coco8-dota.yaml', epochs=3) # train the model
model('https://ultralytics.com/images/bus.jpg') # predict on an image
Appendix#
Additional content below.
# Pip install from source
!pip install git+https://github.com/ultralytics/ultralytics@main
# Git clone and run tests on updates branch
!git clone https://github.com/ultralytics/ultralytics -b main
%pip install -qe ultralytics
# Run tests (Git clone only)
!pytest ultralytics/tests
# Validate multiple models
for x in 'nsmlx':
!yolo val model=yolov8{x}.pt data=coco.yaml